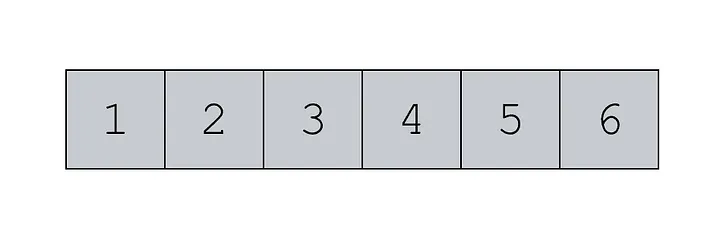
Given an array of integers arr, return true if the number of occurrences of each value in the array is unique or false otherwise.
Example 1:
Input: arr = [1,2,2,1,1,3]
Output: true
Explanation: The value 1 has 3 occurrences, 2 has 2 and 3 has 1. No two values have the same number of occurrences.
Example 2:
Input: arr = [1,2]
Output: false
Example 3:
Input: arr = [-3,0,1,-3,1,1,1,-3,10,0]
Output: true
Constraints:
- 1 <= arr.length <= 1000
- -1000 <= arr[i] <= 1000
Implementation
Python
from collections import Counter
class Solution:
def uniqueOccurrences(self, arr: List[int]) -> bool:
counter = Counter(arr)
seen = set()
for freq in counter.values():
if freq in seen:
return False
seen.add(freq)
return True
Typescript
function uniqueOccurrences(arr: number[]): boolean {
const counter: Map<number, number> = new Map();
const seen: Set<number> = new Set();
for (const num of arr) {
counter.set(num, (counter.get(num) || 0) + 1);
}
for (const freq of counter.values()) {
if (seen.has(freq)) {
return false;
}
seen.add(freq);
}
return true;
}
Go
func uniqueOccurrences(arr []int) bool {
counts := make(map[int]int)
seen := make(map[int]bool)
for _, num := range arr {
counts[num]++
}
for _, count := range counts {
if seen[count] {
return false
}
seen[count] = true
}
return true
}
Overall Logic
The problem asks us to determine if the frequencies of elements in an array are unique. Here’s the general process:
👉Count Occurrences: We need to figure out how many times each number appears in the array.
👉Extract Frequencies: We extract the counts (frequencies) we just calculated.
👉Check for Uniqueness: We then verify if all the extracted frequencies are distinct (no duplicates).
Python
🐍Python’s collections.Counter is a specialized dictionary designed for counting occurrences. It makes step 1 (counting occurrences) very concise.
🐍Counter(arr) does all the counting for us.
🐍Then, we iterate through counter.values() (the frequencies) and use a set to check for uniqueness.
🐍Python’s Counter provides a convenient abstraction that simplifies the counting step, making the Python code more compact.
Go and TypeScript
👓These languages don’t have a built-in Counter equivalent.
👓Therefore, we manually create a map (Go) or Map (TypeScript) to store the counts.
👓The logic for iterating through the counts and checking for uniqueness using a set (TypeScript) or map (Go) is essentially the same as in Python.
If you liked this content I’d appreciate an upvote or a comment. That helps me improve the quality of my posts as well as getting to know more about you, my dear reader.
Muchas gracias!
Follow me for more content like this.
X | PeakD | Rumble | YouTube | Linked In | GitHub | PayPal.me | Medium
Down below you can find other ways to tip my work.
BankTransfer: "710969000019398639", // CLABE
BAT: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875",
ETH: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875",
BTC: "33xxUWU5kjcPk1Kr9ucn9tQXd2DbQ1b9tE",
ADA: "addr1q9l3y73e82hhwfr49eu0fkjw34w9s406wnln7rk9m4ky5fag8akgnwf3y4r2uzqf00rw0pvsucql0pqkzag5n450facq8vwr5e",
DOT: "1rRDzfMLPi88RixTeVc2beA5h2Q3z1K1Uk3kqqyej7nWPNf",
DOGE: "DRph8GEwGccvBWCe4wEQsWsTvQvsEH4QKH",
DAI: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875"