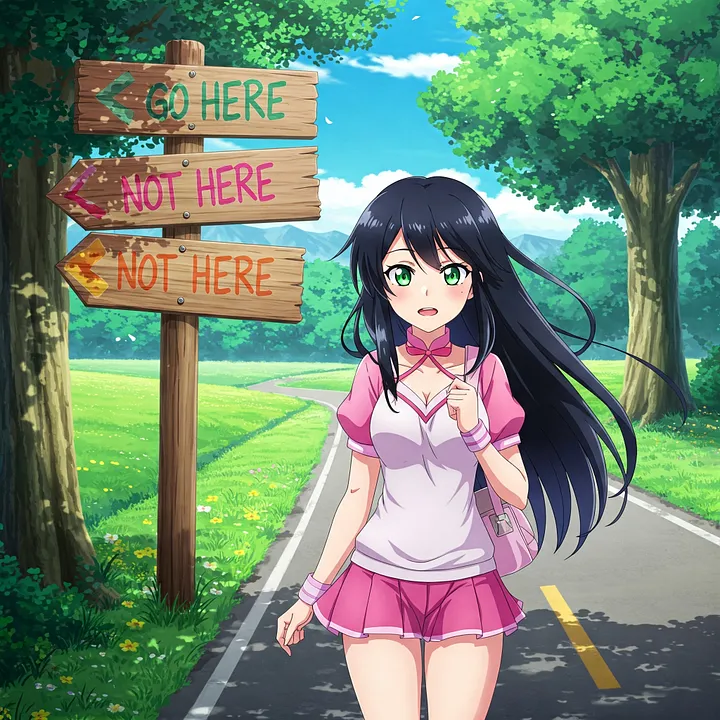
When websites seem to magically transport you from one place to another without you doing anything, that’s the power of redirects at work! Today, I’ll break down three essential types of redirects in a way that’s so simple, even a 5-year-old could understand them. Whether you’re a seasoned developer or just starting your tech journey, understanding these concepts will help you navigate the web more effectively.
🤔What Are Redirects and Why Should You Care?
Have you ever wondered what happens when you type an old web address and somehow end up at a completely different website? That’s a redirect in action! Think of redirects like friendly guides pointing you in the right direction when what you’re looking for has moved.
Redirects are like the helpful staff at a grocery store. When you ask where to find the cereal, they don’t just stare at you blankly — they point you to aisle 7. On the internet, redirects do the same job!
Why should you care about redirects? Well, they:
- Make sure visitors find your content even when it moves
- Keep your website’s search engine rankings when you reorganize
- Create seamless user experiences during website changes
- Help manage traffic across complex systems
Now, let’s explore the three main types of redirects that make the internet work smoothly!
➡️Application Redirects: Your App as a Traffic Controller
🧑💻What Is an Application Redirect?
Imagine you’re playing with toy cars. You’re the traffic controller deciding which car goes where. That’s exactly what your application does with application redirects!
When someone visits your website and clicks a button or logs in, your application might need to send them somewhere else. This happens directly in your application’s code — it’s like your app saying, “Hey, you should go over there now!”
🧑💻How Do Application Redirects Work?
Let’s look at a simple Express.js example:
// This is a simple Express application with redirects
const express = require('express');
const app = express();
// When someone tries to access the homepage
app.get('/', (req, res) => {
// Check if the user is logged in (simplified example)
const isLoggedIn = req.cookies.loggedIn === 'true';
if (isLoggedIn) {
// If they're logged in, send them to the dashboard
// This is our application redirect!
res.redirect('/dashboard');
// Notice how the app itself is making the decision
} else {
// Otherwise, show them the login page
res.render('login');
}
});
// Handle the login form submission
app.post('/login', (req, res) => {
// After successful login, redirect to dashboard
// Another application redirect!
res.redirect('/dashboard');
// The app is saying "now go here instead"
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
Have you noticed how the application is making all the decisions? That’s the key characteristic of application redirects — they happen directly in your code and respond to user actions.
🧑💻When Should You Use Application Redirects?
Application redirects are perfect for:
- After a user submits a form
- When users log in or out
- Creating step-by-step user flows
- Handling authentication checks
- Navigating between different parts of your application
Ever clicked “Submit” on a form and found yourself on a “Thank You” page? That’s an application redirect working its magic!
➡️Service Redirects: The Behind-the-Scenes Traffic Managers
🖧 What Is a Service Redirect?
Now let’s imagine a busy restaurant. Customers don’t talk directly to the chefs — they give orders to waiters who then go to the right chef. If the pasta chef is too busy, the waiter might take your order to a different chef without you ever knowing. That’s a service redirect!
Service redirects happen at a higher level than application redirects. They manage how traffic flows between different services in your infrastructure, often without users or even your application knowing about it.
🖧 How Do Service Redirects Work?
Modern applications are often built as collections of smaller services (microservices). These services might change locations, get updated, or be replaced entirely. Service redirects help manage this complexity.
Let’s look at how you might configure this using Traefik, a popular service proxy:
# Traefik configuration file (simplified)
# Define our services
http:
services:
# Our original payment service
payment-service-v1:
loadBalancer:
servers:
# This service runs on these servers
- url: "http://payment-v1:3000"
# Our new improved payment service
payment-service-v2:
loadBalancer:
servers:
# The new version runs on these servers
- url: "http://payment-v2:3000"
# Define our routing rules
routers:
# The router for our payment API
payment-api:
rule: "PathPrefix(`/api/payments`)"
# Here's the SERVICE REDIRECT - sending traffic to v2 instead of v1
# Users and apps calling this API don't know this is happening!
service: payment-service-v2
# We could even set up a rule to send some traffic to v1 and some to v2
payment-api-split:
rule: "PathPrefix(`/api/payments`) && Headers(`X-Version`, `test`)"
service: payment-service-v1
# This sends requests with a special header to the old service
In this example, all requests to /api/payments are automatically directed to our new payment service (v2). The applications making API calls don't need to change anything - the redirect happens invisibly at the service level.
🖧 When Should You Use Service Redirects?
Service redirects shine when:
- Deploying new versions of services without downtime
- Implementing blue/green deployments
- Setting up canary releases to test new features
- Managing microservice architectures
- Handling service discovery in cloud environments
Have you ever used an app during an update and didn’t notice anything change? Service redirects were probably working behind the scenes!
➡️DNS Redirects: The Internet’s Global Address Book
🌐What Is a DNS Redirect?
Imagine if your family moved to a new house. If someone sends a letter to your old address, the post office might forward it to your new home. DNS redirects work just like that!
DNS (Domain Name System) is like the internet’s address book. A DNS redirect happens when you set up rules at the DNS level to send traffic from one domain to another.
🌐How Do DNS Redirects Work?
Let’s say you’ve moved your website from old-site.com to new-site.com. You want anyone who visits the old site to automatically end up at the new one. Here’s how you might set this up in AWS Route53:
// Route53 DNS configuration (conceptual representation)
{
"RecordSets": [
{
// This record is for old-site.com
"Name": "old-site.com",
"Type": "A",
// Instead of pointing to an IP address, we're using an ALIAS
// This is our DNS redirect!
"AliasTarget": {
// Send all traffic to our new domain
"DNSName": "new-site.com",
"EvaluateTargetHealth": true
// When someone types old-site.com, they'll end up at new-site.com
}
},
{
// We can also redirect a subdomain
"Name": "blog.old-site.com",
"Type": "CNAME",
// CNAME is another way to create a DNS redirect
"TTL": 300,
"ResourceRecords": [
{"Value": "new-blog.new-site.com"}
// blog.old-site.com visitors will go to new-blog.new-site.com
]
}
]
}
DNS redirects are special because they happen before a user even reaches your server. The DNS system itself handles the redirection, making it the earliest type of redirect in our list.
🌐When Should You Use DNS Redirects?
DNS redirects are perfect for:
- Moving your website to a new domain
- Directing subdomains to different services
- Creating vanity URLs (like short.link that goes to reallylong.website)
- Managing regional variations of your website
- Setting up brand protection domains
Have you ever typed in a website address and noticed the URL changed in your browser before the page even started loading? That was likely a DNS redirect!
Putting It All Together: Choosing the Right Redirect for Your Needs
So, when should you use each type of redirect? Let’s make it simple:
🧑💻Use Application Redirects when:
- You’re handling user actions within your application
- You need to redirect based on user-specific conditions
- You’re managing login/logout flows
- You want to redirect after form submissions
🖧 Use Service Redirects when:
- You’re managing traffic between microservices
- You’re updating services without downtime
- You need to split traffic between different versions
- You’re implementing complex service architectures
🌐Use DNS Redirects when:
- You’re changing domain names
- You’re setting up subdomains to point to different services
- You need redirects to happen before requests reach your servers
- You’re managing multiple domains for the same content
Think about redirects like a chain of helpers. DNS redirects are the first helpers you meet, service redirects are the middle managers, and application redirects are the final guides that take you exactly where you need to go.
Conclusion
Understanding these three types of redirects gives you powerful tools for managing your digital world. Whether you’re building a personal website or managing complex systems, knowing which redirect to use and when will make your life easier.
Remember:
- Application redirects happen within your application code
- Service redirects happen at the service discovery level
- DNS redirects happen before requests even reach your infrastructure
Each type has its place in your toolkit, and using them effectively is part of becoming a redirect master.
Next time you click a link and magically arrive somewhere else, you’ll know there’s nothing magical about it — just one of these three types of redirects doing its job perfectly!
What redirect challenges have you faced in your own projects? Do you have questions about implementing any of these redirect types? I’d love to hear from you in the comments!
Happy redirecting!
If you liked this content I’d appreciate an upvote or a comment. That helps me improve the quality of my posts as well as getting to know more about you, my dear reader.
Muchas gracias!
Follow me for more content like this.
X | PeakD | Rumble | YouTube | Linked In | GitHub | PayPal.me | Medium
Down below you can find other ways to tip my work.
BankTransfer: "710969000019398639", // CLABE
BAT: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875",
ETH: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875",
BTC: "33xxUWU5kjcPk1Kr9ucn9tQXd2DbQ1b9tE",
ADA: "addr1q9l3y73e82hhwfr49eu0fkjw34w9s406wnln7rk9m4ky5fag8akgnwf3y4r2uzqf00rw0pvsucql0pqkzag5n450facq8vwr5e",
DOT: "1rRDzfMLPi88RixTeVc2beA5h2Q3z1K1Uk3kqqyej7nWPNf",
DOGE: "DRph8GEwGccvBWCe4wEQsWsTvQvsEH4QKH",
DAI: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875"